Buggy Code in Java and C++ Programming - How to Prevent it?
13 September 2021 5 min read
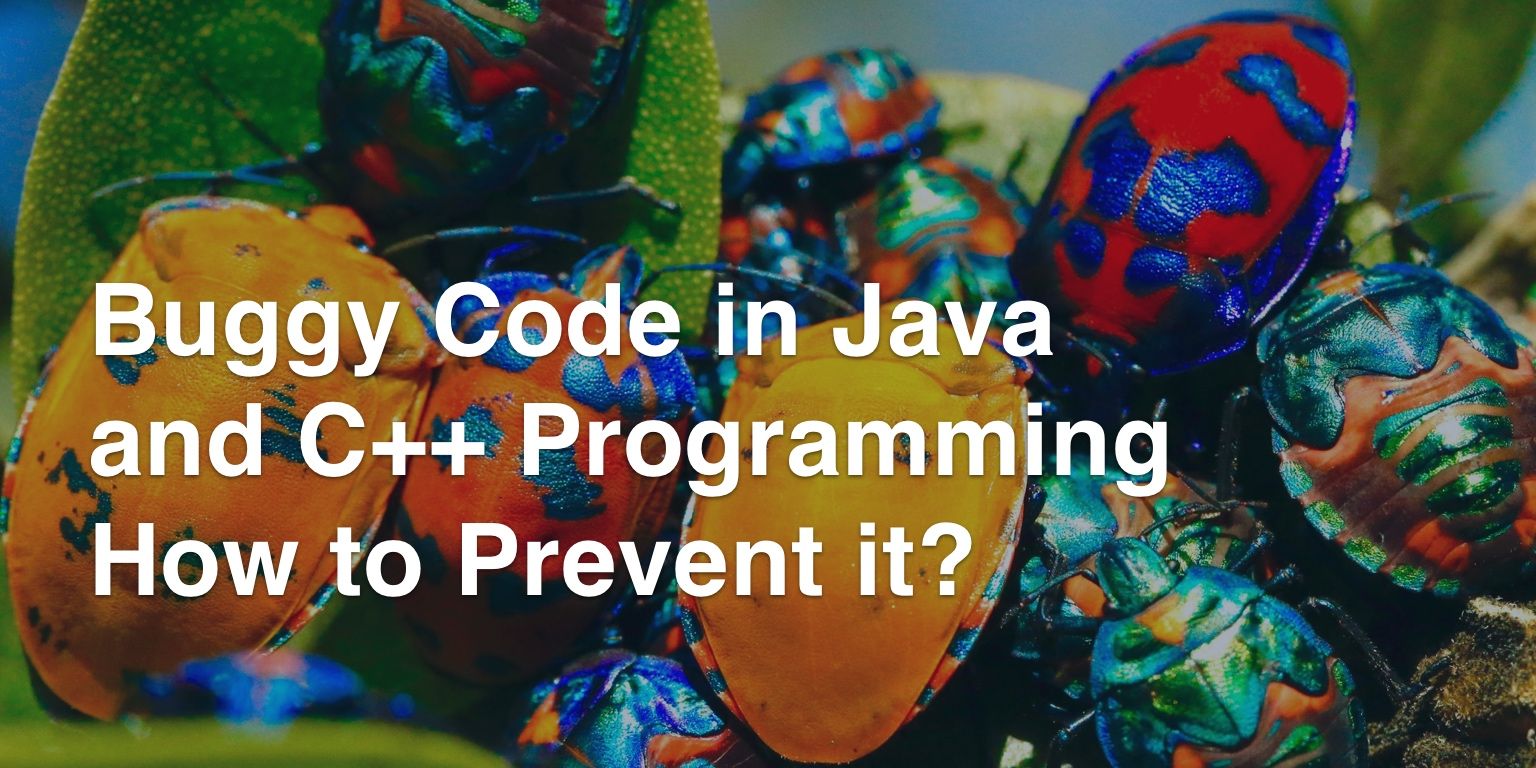
If you have a website or an online application, one of the things that you will run into is buggy code. It's a fancy term for coding that either works in a specific way or doesn't work at all. When you have this type of issue, you will have to fix it. Make sure that your customers can access the information on your site. It can be very time-consuming, so you might as well learn what the common buggy code problems are. It allows you to find and fix bugs as soon as possible.
Buggy code occurs in both languages, and it causes problems for programmers everywhere. In Java, it can cause all kinds of issues. These include performance issues, security issues, and user interface issues. In C++, the language standard itself depends upon strict compliance with the Coding Standards.
REASONS WHY CODE IS BUGGY?
1.Hasty Coding Practices:
Meeting the deadlines, programmers often compromise on the quality of code. To avoid the formation of buggy code, always take time in the coding process to write as much neat code as possible.
2.Poor Documentation:
Documentation errors result in buggy code. The best software engineers will try to document all logged inputs while on the debug monitor. It is one of the most common reasons why code is buggy.
3.Lack of Testing:
Not testing code frequently results in buggy code. While programmers build a program, they employ testing procedures that include comprehensive randomness generation and testing randomness. Software testers know how to do this; they routinely do it to ensure that the source code is as stable and well tested as possible.
How to Write Less Buggy Code?
When you write a piece of code, you are asking for trouble. Bugs are not fun to anyone. They are not something you want when trying to build a program or service. Fortunately, there are some tricks and tips that can help you avoid writing buggy code.
1.Make code more static:
First off, if you want your program or service to be fewer buggies, you need to make sure that the code is as static as possible. Static typing means that programmers will write each code the same way. This is done by typing the variable's name or the expression at the very last line. There are two reasons why this is so important.
First, static typing means that you do not have to remember how to write less buggy code. Instead, you will type in an expression, and the computer will automatically figure out what you need to register.
Secondly, static typing makes it easy to write bug-free code. The reason is that programmers will catch all types of bugs at compile time. Compiling the program or service at compile-time ensures that you don't have to learn how to write buggy code.
2.Write in Patches:
Experienced programmers will prefer to write a patch instead of reproducing the bug through the process described above. One way to achieve this is to make a patch based on a previously undetected but exploitable buffer overflow. Another method is to use in-line clone detection. With this method, the programmer watches for the stack overflow of a buffer, leading to segfaults and other serious problems.
Read more about how to identify what’s causing SegFaults?
3.Use IOP Algorithm:
Some programmers might prefer to use an in-order-of-prime (IOP) algorithm to search through the bug database for buggy lines in code. The IOP algorithm searches through each bit as a binary tree and then checks each pair against each other. While it does not check for every possible input, it is fast and efficient. However, many programmers do not like to spend their time testing this method. They feel it does not consider the non-determinant nature of real-time programs.
4.Test for Bugs:
On the other hand, some programmers may feel that they can prevent bugs from being created. It is why testing for bugs is so important, especially for large projects. In addition to the traditional forms of bug detection such as fuzzing, static analysis, and in-order-of-prime (IOP) tree searching, today's computer scientists employ clone detection and vulnerability assessments. These ensure the safety and security of a software system. When using the techniques of these two tests, programmers can isolate bugs and address specific issues with great precision.
TESTING STRATEGIES THAT WORK BEST TO AVOID BUGGY CODE:
Now, let's talk about a typical testing strategy. A good testing strategy will work for both novice and experienced programmers. Most of the time, there are bugs in the code that will cause the program to behave unexpectedly. Appropriate testing helps you find those bugs early.
1.Unit Testing:
The best strategy for beginners is unit testing, where you test the output of n-dimensional transformations. Unit testing is the act of making sure that your program functions well as one unit. It means that it fills the expected conditions. For example, you should assume that every function is non-local, but otherwise, it may fail the unit test.
The most common type of unit test is the exponential function because it is straightforward to follow. This type of test will give you results for all inputs with 100 million years or more. If a bug occurs, you should be able to extract the leading cause of the bug and the n-1 time complexity of its evolution.
Read more about Unit Testing Techniques
2.Functional Testing:
On the other hand, functional testing involves asking programmers to verify whether or not a piece of code is performing its expected functionality. Often, functional bugs show up as bugs in other parts of the code. The reason is that the programmers have not understood the behavior to the fullest extent possible. They have written many lines of code that don't make any sense. But they don't thoroughly test because it happens so often. This type of bug is more complex to find than the non-functional ones, but it is still worth seeing.
Read more about Functional Testing-Types and Example
3.Test Doubles:
Programmers use test doubles for functional tests of Java code. While they can detect bugs of any type, programmers are sometimes hesitant to use them. They think that they can spot bugs of all kinds - they can't!
Programmers can fix a lot of the bugs found by test doubles. But even if they find and fix a bug quickly, they will usually still have spent several hours debugging the code, and the program will be unusable for a while.
4.Code Duplication:
While the underlying source code of a Java program is almost indeed well-tested, they may need code duplication. It is sometimes necessary for experienced programmers to duplicate a portion of the code to find and document errors.
Moreover, code duplication can result in problems if programmers discover it before a C++ Standard is released. Programmers may not be able to wait for the C++ standard to be completed and released. It helps them eliminate the potential for these kinds of problems. Consequently, when bugs are visible, software engineers must fix them as soon as possible.
Read more about How to avoid software bugs?
BOTTOM LINE:
The truth is, it only takes two people to find one or more bugs in the programming code. Â It takes one person with good programming skills and experience to find, and fix a bug that has taken months to develop. Programmers should permanently correct bugs, and if they are not, the logs should be cleaned and interpreted. All information about a bug should be documented, including its severity.
When debugging grows too tedious, software developers may hire outside technical personnel to assist them. It ensures better code quality and less work for the team.
Updated on the 1st of November 2021